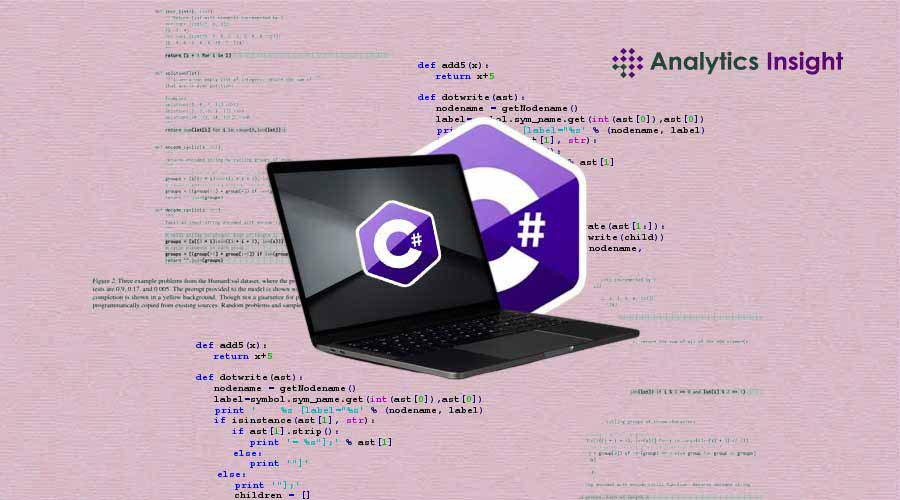
C# is a dynamic and at the same time flexible language driving a myriad of applications under the umbrella of the .NET framework. C# has been designed with strong features promoting efficiency among developers, easing their coding process, and hence coming up with software solutions that are easier to use and maintain.
In this article, we will introduce the top 10 features of C# which every .NET developer must be aware of. We'll break each feature down into an explanation so simple that even if you're a complete novice to C# or programming in general, you get a pretty fair idea of how these tools can help in your coding projects.
LINQ is a powerful feature in C# supporting developers' work in handling data intuitively understandable and readable. Suppose you operate on big data, like a spreadsheet with customer information. One of the powers of LINQ is that it enables filtering, sorting, or any kind of grouping operation within your C# code. It is somehow similar to writing simple queries in a database or Excel spreadsheet but now part of your programming logic.
For example, to get all the customers in a certain city, LINQ has made it possible to write a query in C# that retrieves the information for you without having to go through the data manually. Integration makes working with data so much easier because you are using one language for both querying and processing your data.
Responsiveness is key in any modern application. C#'s Async and Await are a set of features designed to handle operations that take time to complete-such as downloading files or querying a database without hindering the smooth operation of your application. Using Async and Await will let your application do other things when waiting for these operations to finish.
It's like real-world multitasking. While it is still on 'hold up' downloading that file, your computer can still open applications and do some other stuff. Similarly, Async and Await allow an application to run several other processes while waiting for the original action to finalize without freezing or lagging, hence making interaction smoother.
Pattern Matching is a way to easily extend how your code handles and understands various kinds of data. It allows the user to specify a pattern and verify if the data matches that pattern. These can be useful in cases when there are complicated data structures or when there is a need to discriminate between a set of varied types of data.
Suppose you want to teach your program the difference between various shapes. Using pattern matching, you could specify that some chunk of data should be processed one way if it has the pattern of a square and another if it has the pattern of a circle. This feature can highly simplify handling data while also reducing the need to write many conditional statements, and that would make your code look quite neat and read-friendly.
Records are a new feature designed to make it easier to create and work with immutable objects that cannot be changed after they are created. A record defines the template for data structures that remain constant. For example, you can have one record type definition for a customer but create many instances of this record type without changing the actual type definition.
This is helpful when one needs to ensure that the data does not change in the course of the program. It's just like using a template for a business card to generate other cards: the template remains the same, but each card bears different information. Records are important in maintaining data integrity and ensuring that objects retain their values throughout the application life cycle.
Nevertheless, treating null values in programming is the biggest problem for the appearance of errors and crashes. Nullable Reference Types help solve these problems through ways of explicitly defining if a variable of the reference type can have the assigned null value, taking better care about and managing null values at a compile time stage to prevent general runtime errors.
Suppose you have a box, and you want to fill that box with things. Nullable Reference Types enable indicating whether the box can be empty or if it must always contain something. You elude the more common pitfalls of null references by clearly stating that a variable can or cannot be null, and this in itself allows your code to be far more robust and less error-prone.
Tuples allow you to compile several associated pieces of information into a simple, lightweight data structure. Unlike defining some complicated class or object, tuples allow you to package multiple values into one and return them from a function or method, easily.
For example, if a function needs to return the name of a customer along with a number representing an order, a tuple can be used to package these two pieces of information together. This is like receiving one package that contains many items inside of it instead of receiving items one at a time. Tuples provide a straightforward way to handle more than one value without adding in extra complexity.
Expression-bodied members allow lambda expression for properties, methods, and others. It enables writing concise and readable code. This contributes to simplifying your code; it saves your time from going into verbose syntax which will make your code easier to write and maintain.
Imagine taking shorthand notes instead of writing out long paragraphs. Expression-bodied members allow you to do much the same thing but with less code. Your intent is clearer, and your codebase is cleaner. This is especially nice for the case of simple properties and methods where a full implementation would be unduly verbose.
Extension methods are a feature that allows adding new functionality to existing types without having to modify the original and underlying code of those types. This is very useful in situations when one operates with libraries or classes which, for some reason or another, cannot be changed directly.
Think of this like you're adding new features to a tool without actually changing the tool itself. If, for example, you wanted to add a new method to someone else's library class, you could provide that extra functionality using an extension method. What you are doing, then, is extending the functionality of the class while still keeping its original definition intact.
The default methods of an interface can be used to add new methods to an interface without breaking the existing implementations of that interface. This becomes a major enhancement in how interfaces evolve with time, adding more flexibility to backward compatibility.
Think of it like an operating system or software that gets updated for new features, but a user doesn't have to change out their hardware to get these new features. The Default Interface Methods just ensure that code that was written previously continues to function while interfaces that the code targeted get updated for new features.
The global directives make life easier by reducing the number of common namespaces or libraries declared in one place that could be used throughout your project. This feature minimizes the use of repetitive directives at the top of each file and cleans your code, hence making it more maintainable.
Now, imagine setting up a workspace with everything at your fingertips. Global Using Directives do something similar, making sure the commonly used libraries automatically include the project without the developer needing to focalize his efforts elsewhere, amidst all the tedious redundancies.
Mastering these top 10 features of C# will take your development experience to the next level in the .NET framework. These include simplifying data queries with LINQ to managing asynchronous operations with Async and Await, which will make your coding efficient and effective. Familiarize yourself with these tools, and you will be able to craft better, more reliable applications while upping the ante on your overall productivity bar as a .NET developer.
1. What is LINQ in C#?
LINQ (Language Integrated Query) allows you to query and manipulate data directly in C# code, similar to how you handle data in Excel. It simplifies filtering, sorting, and processing data from arrays, collections, or databases, making code more readable and maintainable.
2. How do async and await improve application performance?
Async and Await enable asynchronous programming, allowing tasks to run in the background without freezing the main thread. This keeps applications responsive during long operations, like file downloads or database queries, by handling these tasks efficiently and ensuring a smooth user experience.
3. What are records in C#?
Records in C# are immutable data structures, meaning their values can't be changed after creation. They simplify creating and managing unchangeable objects, offering features like value-based equality and built-in methods for comparison, making them ideal for data transfer objects or configuration settings.
4. What are extension methods in C#?
Extension methods allow you to add new methods to existing types without modifying their original code. This feature is useful for enhancing the functionality of types from external libraries. You define extension methods in a static class, and they appear as if they are part of the original type.
5. What are global using directives in C#?
Global using directives simplifies code management by automatically including specified namespaces across an entire project. This reduces the need for repetitive statements in each file, streamlining code and improving maintainability by centralizing common namespace declarations.