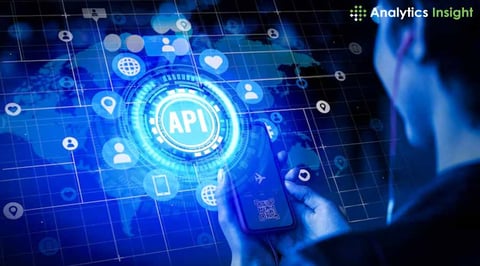
OAuth is an open framework for authorization that authorizes applications to obtain access to protected resources on behalf of a user without ever obtaining the user's credentials. It forms the backbone of modern API security, giving safe and scalable access to data and services. This article guides you on how to build secure APIs using OAuth.
OAuth is based on the system of authentication used for the device's token. On the other hand, if a third-party application would like to access a user's data, the user typically authorizes it just to get an access token that eventually can be used to reach the protected resources.
The general steps that constitute the OAuth flow are usually as follows:
Request for Authorization: An authorization request is made to the authorization server to grant access to a user's data to an application.
User Authorization: The user is shown a consent screen in which he or she can accept or reject whatever request the application has.
Issuance of the Token: When consent is received, an access token is granted to the application by the authorization server on behalf of the user.
Accessing Resources: It relies on an access token to make requests to a resource server, as a stand-in for the user, for protected resources.
Here are some of the grant types defined under OAuth up-to-date, each purposed for different intents:
Authorization Code Grant: The most common grant type, utilized for web applications. A three-legged flow where the user consents to an intermediary authorization server, which in turn returns an access token to the application.
Implicit Grant: Suitable to SPAs, in this grant the authorization server directly returns the access token to the application.
Client Credentials Grant: This grant is used for machine-to-machine authentication where the application requests an access token by using its client credentials directly.
Password Grant: This is used to obtain an access token by supplying the username and password of the user; this grant is not recommended to be used by the general application because of security reasons.
Refresh Token Grant: This grant is used to get a new access token for the already in-use one by using the refresh token issued earlier without repeating the user's login.
Register Your Application: An application is to be created at the Authorization Server, besides gaining the Client Credentials, Client ID, and Client secret.
Handle Authorization Requests: You'll implement a system that will send users for authorization to the consent screen of the authorization server.
Exchange Authorization Code for Access Token: This consent by the user is what the authorization server uses to redirect him/her back to your application with an authorization code. You will make use of this code to buy an access token.
Validate Access Tokens: Before giving access to any resource behind you, we are going to run validation on the access token. The first thing we will do is check its validity and then verify its scope.
Implementation for refresh token handling: Enable an application to obtain new access tokens using the refresh token, if used.
Token Security: Store access tokens securely; do not disclose in cleartext. Initiate HTTPS and safe storage mechanisms.
Scope Management: Define the scope for an application diligently because the scope will inherently constrain the privileges.
Refresh Token Security: Store and manage refresh tokens securely; set an expiration time for refresh tokens so that hijacking an access token cannot be performed.
Rate Limiting: Integrate rate limiting to avoid abuse and protect your API from attacks.
Regular Updates: Keep track of the latest standards related to OAuth and numerous other security best practices.
There are several libraries and frameworks as well that may aid you in implementing OAuth inside of your API seamlessly, leaving you to focus strictly on the business logic. A few popular ones would undoubtedly be:
OAuth 2.0 Provider: A flexible and extensible framework for building OAuth 2.0 providers in PHP.
Spring Security OAuth: A Spring Security module for integrating OAuth 2.0 into Spring-based applications.
OAuth is a very strong mechanism for securing APIs and securely granting access to protected resources. By understanding flow, grant types, and security considerations, OAuth can be correctly implemented to secure your API and the data of your users.
1. What is OAuth?
OAuth is used as an authorization protocol in many applications so that they can easily access resources, which otherwise would be a matter of a user's credentials for getting them safely. It is the cornerstone of modern security for APIs.
2. How does OAuth work?
OAuth uses token-based authentication, where the user authorizes an application to retrieve an access token that can be used later to access the protected resource.
3. What are the various grant types of OAuth?
There are a couple of grant types that are intended to fit different use cases:
Authorization Code Grant: This is the most common for web applications.
Implicit Grant: for SPAs.
Client Credentials Grant: For machine-to-machine authentication
Password Grant: Not recommended because it's dangerous and has security risks.
Refresh Token Grant: Used when a new access token is required without asking the user to re-authenticate.
4. How do I implement OAuth in my API?
To implement OAuth, you will:
Register your application with the authorization server.
Handle authorization requests.
Exchange authorization code for an access token.
Validate access tokens.
Provide refresh token handling.
5. What are some OAuth security concerns?
Token Security: Store access tokens securely. They should not be passed in the clear.
Management of Access Scope: The scope of access must be close-fisted enough for what permissions an application holds. It must be clear which APIs are going to be used as well.
Security of Refresh Token: Store refresh tokens cautiously; use permissions if required; ensure its lifetime is bounded.
Rate Limiting: Bear abuse using the strategy of rate limiting
Stay Updated: Always stay updated with changing OAuth standards and security practices.
6. Can I use OAuth for public APIs?
OAuth can be used for public APIs, but you might have to consider the special case and adapt implementation and security appropriately.
7. What are some popular OAuth libraries and frameworks?
OAuth 2.0 for Node.js
OAuth 2.0 Provider
Spring Security OAuth
8. Is OAuth suitable for mobile applications?
So yes, OAuth will be good enough for mobile applications. Well, if you ever decide to implement OAuth into mobile apps, there are additional security measures you need to study. And of course, user experience is also crucial.
9. What are the benefits of using OAuth compared to more traditional authentication techniques?
Some of the benefits of OAuth include:
More Secure: The security of OAuth is enhanced by specifying that credentials do not get shared across services.
Flexibility: OAuth can be applied over a wide range of application types and authorization servers.
Scalability: OAuth supports hundreds and thousands of users and applications.
10. Does OAuth have a weak spot?
While it is generally a secure authorization framework, OAuth has some potential weak spots:
Complexity: Life for an implementer of OAuth could be a bit more challenging than other security options.
Third-party provider dependency: If your application depends on a third-party provider for authorization, it might be tied to their reliability and security.
User experience: Without a well-designed authorization flow, adding OAuth will simply add unnecessary complexity from the user's vantage point.